The popup window is very useful in most of the times, you can display a separate content in a popup window, like a login screen. In flutter. The popup window should be a Modal window, so we can use [su_label type=”success”]ModalRoute[/su_label] for easy to create it.
Please find the below step for creating a popup window:
1. Create a popup class and inherit to ModalRoute (popup.dart)
class PopupLayout extends ModalRoute { //ToDo... }
We need to override some properties
@override Duration get transitionDuration => Duration(milliseconds: 300); @override bool get opaque => false; @override bool get barrierDismissible => false; @override Color get barrierColor => bgColor == null ? Colors.black.withOpacity(0.5) : bgColor; @override String get barrierLabel => null; @override bool get maintainState => false;
define some variables for dynamic update the margin and content
double top; double bottom; double left; double right; Color bgColor; final Widget child;
and pass them to the constructor
PopupLayout( {Key key, this.bgColor, @required this.child, this.top, this.bottom, this.left, this.right});
override the [su_label type=”success”]buildPage[/su_label] for create the popup content
@override Widget buildPage( BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation, ) { if (top == null) this.top = 10; if (bottom == null) this.bottom = 20; if (left == null) this.left = 20; if (right == null) this.right = 20; return GestureDetector( onTap: () { // call this method here to hide soft keyboard SystemChannels.textInput.invokeMethod('TextInput.hide'); }, child: Material( // This makes sure that text and other content follows the material style type: MaterialType.transparency, //type: MaterialType.canvas, // make sure that the overlay content is not cut off child: SafeArea( bottom: true, child: _buildOverlayContent(context), ), ), ); } //the dynamic content pass by parameter Widget _buildOverlayContent(BuildContext context) { return Container( margin: EdgeInsets.only( bottom: this.bottom, left: this.left, right: this.right, top: this.top), child: child, ); }
override the [su_label type=”success”]buildTransitions[/su_label] for handle the animation
@override Widget buildTransitions(BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation, Widget child) { // You can add your own animations for the overlay content return FadeTransition( opacity: animation, child: ScaleTransition( scale: animation, child: child, ), ); }
2. Create a popup content class (popup_content.dart)
Because we need to handle the status in the content, so we also need a dynamic content class for handle the popup content, and this class will inherit to [su_label type=”warning”]StatefulWidget[/su_label]
class PopupContent extends StatefulWidget { final Widget content; PopupContent({ Key key, this.content, }) : super(key: key); _PopupContentState createState() => _PopupContentState(); }
pass the dynamic content widget
class _PopupContentState extends State<PopupContent> { @override void initState() { super.initState(); } @override Widget build(BuildContext context) { return Container( child: widget.content, ); }
3. Create a common method to call the popup layout
We can create a common method [su_label type=”info”]showPopup[/su_label] for call the popup layout
showPopup(BuildContext context, Widget widget, String title, {BuildContext popupContext}) { Navigator.push( context, PopupLayout( top: 30, left: 30, right: 30, bottom: 50, child: PopupContent( content: Scaffold( appBar: AppBar( title: Text(title), leading: new Builder(builder: (context) { return IconButton( icon: Icon(Icons.arrow_back), onPressed: () { try { Navigator.pop(context); //close the popup } catch (e) {} }, ); }), brightness: Brightness.light, ), resizeToAvoidBottomPadding: false, body: widget, ), ), ), ); }
for the dynamic content widget, we can use any widgets as below:
Widget _popupBody() { return Container( child: Text('This is a popup window'), ); }
And please find the below sample screen capture for the result:
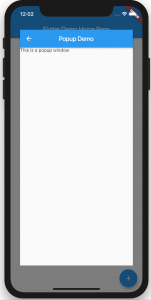
You can find the below full source code for the demo. Because the iOS folder is larger, so I didn’t include it in the source code
coderblog_popup_window.zip (7024 downloads )